1
[Java] Xử lý file căn bản Fri Apr 19, 2013 12:27 am
Admin
Admin
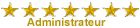
Server:
Client:
- Code:
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.NoSuchElementException;
import java.util.Scanner;
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author nguyenict
*/
public class ExampleFileServer {
public static void main(String[] args) {
try{
ServerSocket ss = new ServerSocket(9999);
System.out.println("Server is running!");
while (true){
Socket s = ss.accept();
System.out.println("Client "+s.getInetAddress() +" is connected!");
exampleClient c = new exampleClient(s);
c.start();
}
}catch(IOException e){
}
}
}
class exampleClient extends Thread{
Socket s;
public exampleClient(Socket s){
this.s=s;
}
@Override
public void run(){
try{
Scanner in = new Scanner(s.getInputStream());
PrintWriter out = new PrintWriter(s.getOutputStream());
while (true){
String recive = in.nextLine();
String[] cmd = recive.split(" ",2);
System.out.println(cmd[0] +" - "+cmd[1]);
File f = new File(cmd[1]);
if (cmd[0].toUpperCase().equals("READ")){
if (!f.exists()){
out.println("NO");
out.flush();
}
else{
out.println("YES");
out.flush();
Scanner file = new Scanner(new File(cmd[1]));
while (file.hasNextLine()){
out.println(file.nextLine());
}
out.println("END");
out.flush();
}
}else
if (cmd[0].toUpperCase().equals("DELETE")){
if (!f.exists()){
out.println("NO");
out.flush();
}
else{
if (f.delete()) out.println("YES");
else
out.println("NO");
out.flush();
}
}else
if (cmd[0].toUpperCase().equals("WRITE")){
if (!f.exists())
f.createNewFile();
FileWriter writeFile = new FileWriter(f, true);
String content;
while (true){
content = in.nextLine();
if (content.equals("END")){
writeFile.close();
break;
}
writeFile.write(content + System.getProperty("line.separator"));
}
}else
if (cmd[0].toUpperCase().equals("CLEAR")){
f.delete();
f.createNewFile();
}
}
}
catch(IOException e){
System.out.println("Loi nhap xuat");
}
catch(NoSuchElementException e){
System.out.println("Client disconnect with Server!");
}
}
}
Client:
- Code:
import java.io.IOException;
import java.io.PrintWriter;
import java.net.Socket;
import java.util.Scanner;
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
/**
*
* @author nguyenict
*/
public class ExampleFileClient {
public static void menu(){
System.out.println("______Menu______");
System.out.println("1. READ FILE");
System.out.println("2. DELETE FILE");
System.out.println("3. WRITE FILE");
System.out.println("4. CLEAR FILE");
System.out.println("Q to quit");
}
public static void main(String[] args) {
try{
Socket s = new Socket("127.0.0.1",9999);
Scanner in = new Scanner(s.getInputStream());
Scanner kb = new Scanner(System.in);
PrintWriter out = new PrintWriter(s.getOutputStream());
while (true){
menu();
String choose="";
while (!choose.equals("1") && !choose.equals("2") && !choose.equals("3") && !choose.equals("4")&& !choose.equals("Q")){
System.out.print("Choose: ");
choose = kb.nextLine();
}
if (choose.equals("Q"))
break;
System.out.print("Enter Path file: ");
String path = kb.nextLine();
if (choose.equals("1")){
out.println("READ "+path);
out.flush();
String ans = in.nextLine();
if (ans.equals("YES")){
System.out.println("This conntent:");
while (true){
String conntent = in.nextLine();
if (conntent.equals("END")){
break;
}
System.out.println(conntent);
}
}
else{
System.out.println("File not exits!");
}
}else
if (choose.equals("2")){
out.println("DELETE "+path);
out.flush();
String ans = in.nextLine();
if (ans.equals("YES"))
System.out.println("Successful!");
else System.out.println("Unsuccessful!");
}else
if (choose.equals("3")){
out.println("WRITE "+path);
out.flush();
System.out.println("type END to finish.");
System.out.print("CONTENT: ");
while (true){
String conntent = kb.nextLine();
out.println(conntent);
out.flush();
if (conntent.equals("END"))
break;
}
}else
if (choose.equals("4")){
out.println("CLEAR "+path);
out.flush();
System.out.println("Clear success!");
}else
if (choose.equals("Q")){
break;
}
}
}
catch(IOException e){
}
}
}